3.5.2.4.4. 验证器控件
Validator
设计用来检查可视化组件中输入的值。
验证和输入检查是需要区分开来的,输入检查是说:假设一个文本组件(比如,TextField)的数据类型设置的不是字符串(这种情况可能出现在绑定实体属性或者手动设置控件的 验证不会在输入同时或者失去焦点时马上反馈,而是会在组件的 |
CUBA 框架包含了一组最常用的验证器实现,可以直接在项目中使用:
在界面的 XML 描述中,组件的验证器可以在嵌套的 validators
元素中定义。
可以通过 CUBA Studio 添加验证器。下面是给 TextField
组件添加验证器的例子:
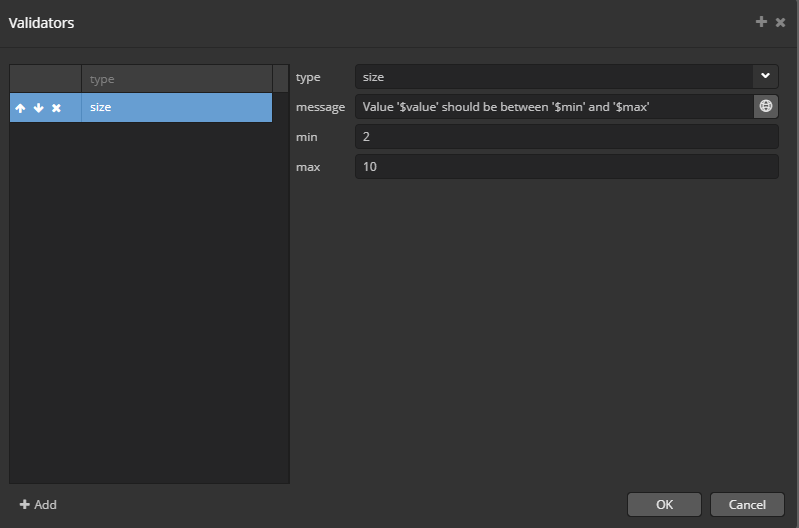
每个验证器都是一个 Prototype Bean
,如果希望在 Java 代码中使用验证器,需要通过 BeanLocator
来获取。
有些验证器在错误消息中使用了 Groovy 字符串。这样的话,可以给错误消息传递参数(比如,$value
)。这些参数会考虑用户的 locale 配置。
你可以使用自定义的 Java 类作为验证器,自定义类需要实现 Consumer
接口。
在界面的 XML 描述中,自定义的验证器可以在嵌套的 validator
元素中定义。
如果验证器是作为内部类实现的话,则需要使用
|
给组件设置验证类的方法除了 XML 描述之外,也可以使用编程的方式 - 使用组件的 addValidator()
方法添加验证器的实例。
创建验证邮编的验证器类:
public class ZipValidator implements Consumer<String> {
@Override
public void accept(String s) throws ValidationException {
if (s != null && s.length() != 6)
throw new ValidationException("Zip must be of 6 characters length");
}
}
在TextField组件中使用邮编验证器的示例:
<textField id="zipField" property="zip">
<validator class="com.company.sample.web.ZipValidator"/>
</textField>
在界面控制器用编程的方式设置验证器:
zipField.addValidator(value -> {
if (value != null && value.length() != 6)
throw new ValidationException("Zip must be of 6 characters length");
});
下面我们看看框架预定义的这些验证器。
- DecimalMaxValidator
-
检查值必须小于等于指定的最大值。 支持的类型:
BigDecimal
、BigInteger
、Long
、Integer
以及使用当前 locale 表示BigDecimal
的String
类型。它有如下属性:
-
value
− 最大值(必须); -
inclusive
− 当设置成true
时,值必须小于或等于指定的最大值。默认值是true
。 -
message
− 自定义的消息,用于在验证失败时展示给用户。该消息可以包含$value
和$max
关键字,用于格式化输出。
默认消息键值:
-
validation.constraints.decimalMaxInclusive
-
validation.constraints.decimalMax
XML 描述中用法:
<textField id="numberField" property="numberProperty"> <validators> <decimalMax value="10000" inclusive="false" message="Value '$value' cannot be greater than `$max`"/> </validators> </textField>
Java 代码用法:
DecimalMaxValidator maxValidator = beanLocator.getPrototype(DecimalMaxValidator.NAME, new BigDecimal(100)); numberField.addValidator(maxValidator);
-
- DecimalMinValidator
-
检查值必须大于等于指定的最小值。 支持的类型:
BigDecimal
、BigInteger
、Long
、Integer
以及使用当前 locale 表示BigDecimal
的String
类型。它有如下属性:
-
value
− 最小值(必须); -
inclusive
− 当设置成true
时,值必须大于或等于指定的最小值。默认值是true
。 -
message
− 自定义的消息,用于在验证失败时展示给用户。该消息可以包含$value
和$min
关键字,用于格式化输出。
默认消息键值:
-
validation.constraints.decimalMinInclusive
-
validation.constraints.decimalMin
XML 描述中用法:
<textField id="numberField" property="numberProperty"> <validators> <decimalMin value="100" inclusive="false" message="Value '$value' cannot be less than `$min`"/> </validators> </textField>
Java 代码用法:
DecimalMinValidator minValidator = beanLocator.getPrototype(DecimalMinValidator.NAME, new BigDecimal(100)); numberField.addValidator(minValidator);
-
- DigitsValidator
-
检查值必须是一个指定范围内的数字。 支持的类型:
BigDecimal
、BigInteger
、Long
、Integer
以及使用当前 locale 表示BigDecimal
的String
类型。它有如下属性:
-
integer
− 整数部分数字的个数(必须); -
fraction
− 小数部分数字的个数(必须); -
message
− 自定义的消息,用于在验证失败时展示给用户。该消息可以包含$value
、$integer
和$fraction
关键字,用于格式化输出。
默认消息键值:
-
validation.constraints.digits
XML 描述中用法:
<textField id="numberField" property="numberProperty"> <validators> <digits integer="3" fraction="2" message="Value '$value' is out of bounds ($integer digits are expected in integer part and $fraction in fractional part)"/> </validators> </textField>
Java 代码用法:
DigitsValidator digitsValidator = beanLocator.getPrototype(DigitsValidator.NAME, 3, 2); numberField.addValidator(digitsValidator);
-
- FutureOrPresentValidator
-
检查日期或时间是否在将来或者现在。它不使用 Groovy 字符串,所以没有参数可用于消息格式化。 支持的类型:
java.util.Date
、LocalDate
、LocalDateTime
、LocalTime
、OffsetDateTime
、OffsetTime
。它有如下属性:
-
checkSeconds
− 当设置成true
时,验证器需要使用秒和毫秒比较日期或者时间。默认值是false
。 -
message
− 自定义的消息,用于在验证失败时展示给用户。
默认消息键值:
-
validation.constraints.futureOrPresent
XML 描述中用法:
<dateField id="dateTimePropertyField" property="dateTimeProperty"> <validators> <futureOrPresent checkSeconds="true"/> </validators> </dateField>
Java 代码用法:
FutureOrPresentValidator futureOrPresentValidator = beanLocator.getPrototype(FutureOrPresentValidator.NAME); dateField.addValidator(futureOrPresentValidator);
-
- FutureValidator
-
它验证时间或者日期必须在将来。它不使用 Groovy 字符串,所以没有参数可用于消息格式化。 支持的类型:
java.util.Date
、LocalDate
、LocalDateTime
、LocalTime
、OffsetDateTime
、OffsetTime
。它有如下属性:
-
checkSeconds
− 当设置成true
时,验证器需要使用秒和毫秒比较日期或者时间。默认值是false
。 -
message
− 自定义的消息,用于在验证失败时展示给用户。
默认消息键值:
-
validation.constraints.future
XML 描述中用法:
<timeField id="localTimeField" property="localTimeProperty" showSeconds="true"> <validators> <future checkSeconds="true"/> </validators> </timeField>
Java 代码用法:
FutureValidator futureValidator = beanLocator.getPrototype(FutureValidator.NAME); timeField.addValidator(futureValidator);
-
- MaxValidator
-
检查值必须小于或等于指定的最大值。 支持的类型:
BigDecimal
、BigInteger
、Long
、Integer
。它有如下属性:
-
value
− 最大值(必须); -
message
− 自定义的消息,用于在验证失败时展示给用户。该消息可以包含$value
和$max
关键字,用于格式化输出。
默认消息键值:
-
validation.constraints.max
XML 描述中用法:
<textField id="numberField" property="numberProperty"> <validators> <max value="20500" message="Value '$value' must be less than or equal to '$max'"/> </validators> </textField>
Java 代码用法:
MaxValidator maxValidator = beanLocator.getPrototype(MaxValidator.NAME, 20500); numberField.addValidator(maxValidator);
-
- MinValidator
-
检查值必须大于等于指定的最小值。 支持的类型:
BigDecimal
、BigInteger
、Long
、Integer
。它有如下属性:
-
value
− 最小值(必须); -
message
− 自定义的消息,用于在验证失败时展示给用户。该消息可以包含$value
和$min
关键字,用于格式化输出。
默认消息键值:
-
validation.constraints.min
XML 描述中用法:
<textField id="numberField" property="numberProperty"> <validators> <min value="30" message="Value '$value' must be greater than or equal to '$min'"/> </validators> </textField>
Java 代码用法:
MinValidator minValidator = beanLocator.getPrototype(MinValidator.NAME, 30); numberField.addValidator(minValidator);
-
- NegativeOrZeroValidator
-
检查值必须小于等于 0。 支持的类型:
BigDecimal
、BigInteger
、Long
、Integer
、Double
、Float
。它有如下属性:
-
message
− 自定义的消息,用于在验证失败时展示给用户。该消息可以包含$value
关键字,用于格式化输出。注意,Float
并没有它自己的数据类型,不会使用用户的 locale 进行格式化。
默认消息键值:
-
validation.constraints.negativeOrZero
XML 描述中用法:
<textField id="numberField" property="numberProperty"> <validators> <negativeOrZero message="Value '$value' must be less than or equal to 0"/> </validators> </textField>
Java 代码用法:
NegativeOrZeroValidator negativeOrZeroValidator = beanLocator.getPrototype(NegativeOrZeroValidator.NAME); numberField.addValidator(negativeOrZeroValidator);
-
- NegativeValidator
-
检查值必须小于 0。 支持的类型:
BigDecimal
、BigInteger
、Long
、Integer
、Double
、Float
。它有如下属性:
-
message
− 自定义的消息,用于在验证失败时展示给用户。该消息可以包含$value
关键字,用于格式化输出。注意,Float
并没有它自己的数据类型,不会使用用户的 locale 进行格式化。
默认消息键值:
-
validation.constraints.negative
XML 描述中用法:
<textField id="numberField" property="numberProperty"> <validators> <negative message="Value '$value' should be less than 0"/> </validators> </textField>
Java 代码用法:
NegativeValidator negativeValidator = beanLocator.getPrototype(NegativeValidator.NAME); numberField.addValidator(negativeValidator);
-
- NotBlankValidator
-
检查值至少包含一个非空字符。它不使用 Groovy 字符串,所以没有参数可用于消息格式化。 支持的类型:
String
。它有如下属性:
-
message
− 自定义的消息,用于在验证失败时展示给用户。
默认消息键值:
-
validation.constraints.notBlank
XML 描述中用法:
<textField id="textField" property="textProperty"> <validators> <notBlank message="Value must contain at least one non-whitespace character"/> </validators> </textField>
Java 代码用法:
NotBlankValidator notBlankValidator = beanLocator.getPrototype(NotBlankValidator.NAME); textField.addValidator(notBlankValidator);
-
- NotEmptyValidator
-
检查值不是
null
也非空。 支持的类型:Collection
和String
。它有如下属性:
-
message
− 自定义的消息,用于在验证失败时展示给用户。该消息可以包含$value
关键字,用于格式化输出,只对String
类型有效。
默认消息键值:
-
validation.constraints.notEmpty
XML 描述中用法:
<textField id="textField" property="textProperty"> <validators> <notBlank message="Value must contain at least one non-whitespace character"/> </validators> </textField>
Java 代码用法:
NotBlankValidator notBlankValidator = beanLocator.getPrototype(NotBlankValidator.NAME); textField.addValidator(notBlankValidator);
-
- NotNullValidator
-
检查值不是
null
。它不使用 Groovy 字符串,所以没有参数可用于消息格式化。它有如下属性:
-
message
− 自定义的消息,用于在验证失败时展示给用户。
默认消息键值:
-
validation.constraints.notNull
XML 描述中用法:
<textField id="numberField" property="numberProperty"> <validators> <notNull/> </validators> </textField>
Java 代码用法:
NotNullValidator notNullValidator = beanLocator.getPrototype(NotNullValidator.NAME); numberField.addValidator(notNullValidator);
-
- PastOrPresentValidator
-
检查时间或者日期在过去或者现在。它不使用 Groovy 字符串,所以没有参数可用于消息格式化。 支持的类型:
java.util.Date
、LocalDate
、LocalDateTime
、LocalTime
、OffsetDateTime
、OffsetTime
。它有如下属性:
-
checkSeconds
− 当设置为ture
时,验证器需要使用秒和毫秒比较日期或者时间。默认值是false
。 -
message
− 自定义的消息,用于在验证失败时展示给用户。
默认消息键值:
-
validation.constraints.pastOrPresent
XML 描述中用法:
<dateField id="dateTimeField" property="dateTimeProperty"> <validators> <pastOrPresent/> </validators> </dateField>
Java 代码用法:
PastOrPresentValidator pastOrPresentValidator = beanLocator.getPrototype(PastOrPresentValidator.NAME); numberField.addValidator(pastOrPresentValidator);
-
- PastValidator
-
检查时间或者日期在过去。它不使用 Groovy 字符串,所以没有参数可用于消息格式化。 支持的类型:
java.util.Date
、LocalDate
、LocalDateTime
、LocalTime
、OffsetDateTime
、OffsetTime
。它有如下属性:
-
checkSeconds
− 当设置为ture
时,验证器需要使用秒和毫秒比较日期或者时间。默认值是false
。 -
message
− 自定义的消息,用于在验证失败时展示给用户。
默认消息键值:
-
validation.constraints.past
XML 描述中用法:
<dateField id="dateTimeField" property="dateTimeProperty"> <validators> <pastOrPresent/> </validators> </dateField>
Java 代码用法:
PastOrPresentValidator pastOrPresentValidator = beanLocator.getPrototype(PastOrPresentValidator.NAME); numberField.addValidator(pastOrPresentValidator);
-
- PositiveOrZeroValidator
-
检查值必须大于等于 0。 支持的类型:
BigDecimal
、BigInteger
、Long
、Integer
、Double
、Float
。它有如下属性:
-
message
− 自定义的消息,用于在验证失败时展示给用户。该消息可以包含$value
关键字,用于格式化输出。注意,Float
并没有它自己的数据类型,不会使用用户的 locale 进行格式化。
默认消息键值:
-
validation.constraints.positiveOrZero
XML 描述中用法:
<textField id="numberField" property="numberProperty"> <validators> <positiveOrZero message="Value '$value' should be greater than or equal to '0'"/> </validators> </textField>
Java 代码用法:
PositiveOrZeroValidator positiveOrZeroValidator = beanLocator.getPrototype(PositiveOrZeroValidator.NAME); numberField.addValidator(positiveOrZeroValidator);
-
- PositiveValidator
-
检查值必须严格大于 0。 支持的类型:
BigDecimal
、BigInteger
、Long
、Integer
、Double
、Float
。它有如下属性:
-
message
− 自定义的消息,用于在验证失败时展示给用户。该消息可以包含$value
关键字,用于格式化输出。注意,Float
并没有它自己的数据类型,不会使用用户的 locale 进行格式化。
默认消息键值:
-
validation.constraints.positive
XML 描述中用法:
<textField id="numberField" property="numberProperty"> <validators> <positive message="Value '$value' should be greater than '0'"/> </validators> </textField>
Java 代码用法:
PositiveValidator positiveValidator = beanLocator.getPrototype(PositiveValidator.NAME); numberField.addValidator(positiveValidator);
-
- RegexpValidator
-
检查
String
的值是否能匹配提供的正则表达式。 支持的类型:String
。它有如下属性:
-
regexp
− 一个用于匹配的正则表达式(必须); -
message
− 自定义的消息,用于在验证失败时展示给用户。该消息可以包含$value
关键字,用于格式化输出。
默认消息键值:
-
validation.constraints.regexp
XML 描述中用法:
<textField id="textField" property="textProperty"> <validators> <regexp regexp="[a-z]*"/> </validators> </textField>
Java 代码用法:
RegexpValidator regexpValidator = beanLocator.getPrototype(RegexpValidator.NAME, "[a-z]*"); textField.addValidator(regexpValidator);
-
- SizeValidator
-
检查值在一定范围内。 支持的类型:
Collection
和String
。它有如下属性:
-
min
− 最小值(不包含),不能小于 0。默认值是 0; -
max
− 最大值(不包含),不能小于 0。默认值是Integer.MAX_VALUE
; -
message
− 自定义的消息,用于在验证失败时展示给用户。该消息可以包含$value
(只对String
类型有效),$min
、$max
关键字,用于格式化输出。
默认消息键值:
-
validation.constraints.collectionSizeRange
-
validation.constraints.sizeRange
XML 描述中用法:
<textField id="textField" property="textProperty"> <validators> <size min="2" max="10" message="Value '$value' should be between '$min' and '$max'"/> </validators> </textField> <twinColumn id="twinColumn"> <validators> <size min="2" max="4" message="Collection size must be between $min and $max"/> </validators> </twinColumn>
Java 代码用法:
SizeValidator sizeValidator = beanLocator.getPrototype(SizeValidator.NAME); textField.addValidator(sizeValidator);
-